Problem Statement:
If the company you work for doesn’t use ServiceNow, or perhaps it does but you are unable to get the needed privilege’s to access it using the SNOW REST API. What to do?
Solution:
The ServiceNow Developer program. Through this program you are given full access to a ServiceNow instance at no cost. You can also use this instance for experimenting with ServiceNow tenant side scripting capabilities.
Required PowerShell Modules:
- ServiceNow: Sam Martin has created a module and published it on GitHub that performs most of the REST API heavy lifting .
Step 1: After you have signed up for the developer program and received access to your developer instance, you should create a user and assign that user these roles:
- rest_api_explorer
- itil (assigning this role will actually assign several roles to the user)
Once you have the roles assigned, test the user using the Impersonate User feature.
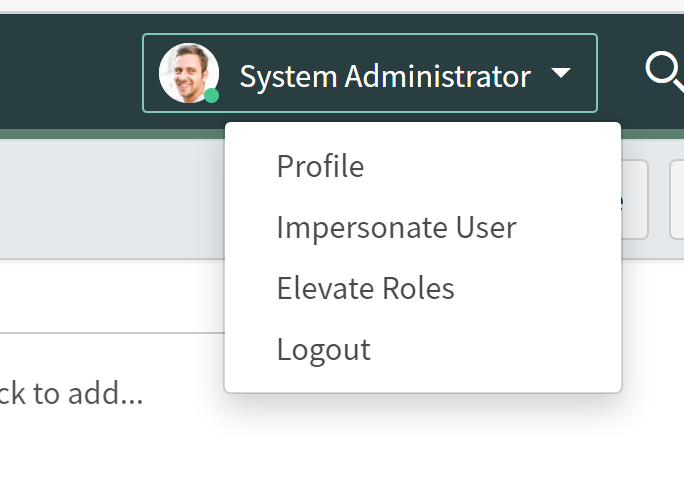
While impersonating your test user perform a search for the REST API Explorer.
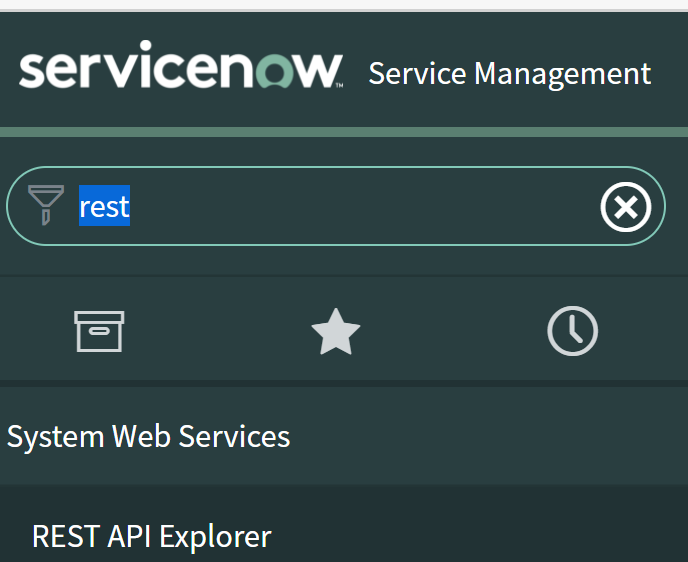
If your test user is able to use the REST API Explorer you are good to go for the next steps.
Open a PowerShell session and import the ServiceNow module. As you can see there are 26 different command provided.
PS C:\Users\chris> Get-Command -Module servicenow
CommandType Name Version Source
----------- ---- ------- ------
Function Add-ServiceNowAttachment 1.8.0 servicenow
Function Get-ServiceNowAttachment 1.8.0 servicenow
Function Get-ServiceNowAttachmentDetail 1.8.0 servicenow
Function Get-ServiceNowChangeRequest 1.8.0 servicenow
Function Get-ServiceNowConfigurationItem 1.8.0 servicenow
Function Get-ServiceNowIncident 1.8.0 servicenow
Function Get-ServiceNowRequest 1.8.0 servicenow
Function Get-ServiceNowRequestItem 1.8.0 servicenow
Function Get-ServiceNowTable 1.8.0 servicenow
Function Get-ServiceNowTableEntry 1.8.0 servicenow
Function Get-ServiceNowUser 1.8.0 servicenow
Function Get-ServiceNowUserGroup 1.8.0 servicenow
Function New-ServiceNowChangeRequest 1.8.0 servicenow
Function New-ServiceNowIncident 1.8.0 servicenow
Function New-ServiceNowQuery 1.8.0 servicenow
Function New-ServiceNowTableEntry 1.8.0 servicenow
Function Remove-ServiceNowAttachment 1.8.0 servicenow
Function Remove-ServiceNowAuth 1.8.0 servicenow
Function Remove-ServiceNowTableEntry 1.8.0 servicenow
Function Set-ServiceNowAuth 1.8.0 servicenow
Function Test-ServiceNowAuthIsSet 1.8.0 servicenow
Function Update-ServiceNowChangeRequest 1.8.0 servicenow
Function Update-ServiceNowIncident 1.8.0 servicenow
Function Update-ServiceNowNumber 1.8.0 servicenow
Function Update-ServiceNowRequestItem 1.8.0 servicenow
Function Update-ServiceNowTableEntry 1.8.0 servicenow
The first two commandlets we need are Set-ServiceNowAuth and TestServiceNowAuth.
PS C:\Users\chris> $url = '<your-test-snow-url>
PS C:\Users\chris> Set-ServiceNowAuth -url $url -Credentials (Get-Credential)
PS C:\Users\chris> Test-ServiceNowAuthIsSet
True
PS C:\Users\chris>
If Test-ServiceNowAuth returns True you have successfully established a session with your developer ServiceNow instance. Now lets create a new Incident.
$Params = @{Caller = "Bugs Bunny"
ShortDescription = "What's Up Doc"
Description = "I knew I should have taken that left turn"
Comment = "Help"
}
New-ServiceNowIncident @Params
In the web interface we can see that the ticket was created.

But as you can see it hasn’t been assigned to anyone yet.. First step to assigning a ticket is to assign the correct user group.
PS C:\Users\chris> get-help Update-ServiceNowIncident
NAME
Update-ServiceNowIncident
SYNTAX
Update-ServiceNowIncident -SysId <string> -Values <hashtable> [<CommonParameters>]
Update-ServiceNowIncident -SysId <string> -Values <hashtable> -Connection <hashtable> [<CommonParameters>]
Update-ServiceNowIncident -SysId <string> -Values <hashtable> -ServiceNowCredential <pscredential> -ServiceNowURL <string>
[<CommonParameters>]
ALIASES
None
REMARKS
None
Looks like I’ll need the sysId for the Incident in order to perform the update.
PS C:\Users\chris> $inc = Get-ServiceNowIncident -MatchContains @{short_description = 'Up Doc'}
PS C:\Users\chris> $inc.sys_id
0a317b2adb342010bbc0a0f2ca961957
Now lets look at the details of the Service Desk user group. The group Name attribute must be unique in ServiceNow so I can match on that.
PS C:\Users\chris> $grp = Get-ServiceNowUserGroup -MatchExact @{Name = 'Service Desk'}
PS C:\Users\chris> $grp | Format-List
parent :
manager : @{display_value=Beth Anglin;
link=https://dev70964.service-now.com/api/now/v1/table/sys_user/46d44a23a9fe19810012d100cca80666}
sys_mod_count : 0
active : true
description :
source :
sys_updated_on : 9/23/2020 8:14:56 PM
sys_tags :
type :
sys_id : d625dccec0a8016700a222a0f7900d06
sys_updated_by : glide.maint
cost_center :
default_assignee :
sys_created_on : 9/23/2020 8:14:56 PM
name : Service Desk
exclude_manager : false
email : [email protected]
include_members : false
sys_created_by : glide.maint
I’m ready to set the group for the Incident record.
Update-ServiceNowIncident -SysId '0a317b2adb342010bbc0a0f2ca961957' -Values @{assignment_group="Service Desk"}
PS C:\Users\chris> $inc = Get-ServiceNowIncident -MatchContains @{short_description = 'Up Doc'}
PS C:\Users\chris> $inc.assignment_group | Select-Object display_Value
display_value
-------------
Service Desk
PS C:\Users\chris>
Now that the group is assigned, select a user from that group to assign the ticket. In order to find the group members using PowerShell you need to know which table(s) to use. In this case there is one table, sys_user_grmember, that has the mapping of users to group.
While the ServiceNow module does not have a cmdlet explicitly for this table, it does have the generic Get-ServiceNowTable.
get-help get-serviceNowTable
NAME
Get-ServiceNowTable
SYNOPSIS
Retrieves records for the specified table
SYNTAX
Get-ServiceNowTable -Table <String> [-Query <String>] [-Limit <Int32>] [-Properties <String[]>] [-DisplayValues <String>]
[-IncludeTotalCount] [-Skip <UInt64>] [-First <UInt64>] [<CommonParameters>]
Get-ServiceNowTable -Table <String> [-Query <String>] [-Limit <Int32>] [-Properties <String[]>] [-DisplayValues <String>]
-Credential <PSCredential> -ServiceNowURL <String> [-IncludeTotalCount] [-Skip <UInt64>] [-First <UInt64>] [<CommonParameters>]
Get-ServiceNowTable -Table <String> [-Query <String>] [-Limit <Int32>] [-Properties <String[]>] [-DisplayValues <String>]
-Connection <Hashtable> [-IncludeTotalCount] [-Skip <UInt64>] [-First <UInt64>] [<CommonParameters>]
$usrs = Get-ServiceNowTable -Table 'sys_user_grmember' -First 1000 | `%{foreach($g in $_.group){if($g.display_value -eq 'Service Desk'){%{($_.user).display_value}}}}
PS C:\Users\chris> $usrs
Beth Anglin
David Loo
ITIL User
PS C:\Users\chris>
Looks like there are three users to choose from. I’ll go with Beth Anglin.
Update-ServiceNowIncident -SysId '0a317b2adb342010bbc0a0f2ca961957' -Values @{assigned_to="Beth Anglin"}
As you can see below the assigned to field has been updated and the note added as well.

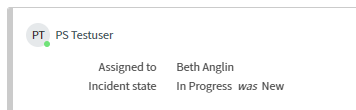